Python,
Turbocharged
Exaloop’s Codon framework compiles Python to native machine code for 10-100x performance gains, multithreading, GPU acceleration & more. Get C performance while staying in Python.
Taking Python to the next level
Exaloop develops tools that empower anyone with basic Python programming experience to write scalable code.
Python simplicity, C performance
Benchmark (speedup over Python)
Benchmarks are run on the following setups:
- 2021 16-inch MacBook Pro, Apple M1 Max, 64GB RAM, macOS Monterey 12.5.1
- Python: 3.10.8
- PyPy: 7.3.9 nightly build 2022-11-02, 106335-024a5669d75d
- Clang: Apple clang version 13.1.6
- Codon: 0.15.0, commit 5480050b0ae3fb39b30e3a71df260ce5e91e5064
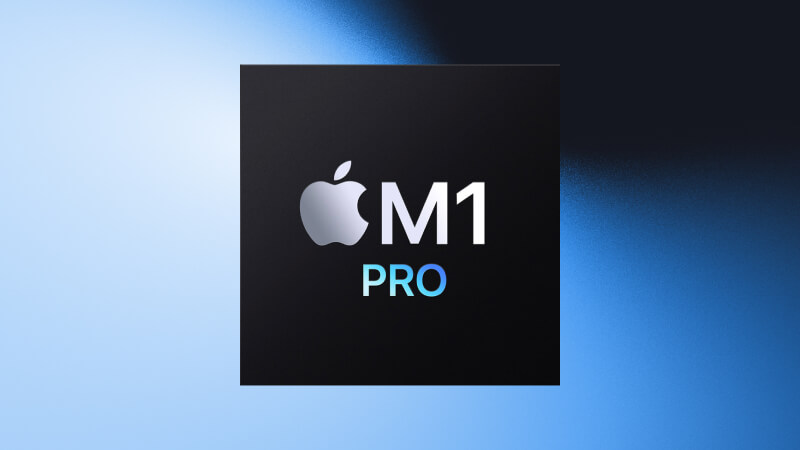
- CentOS Linux 7 (Core), 64 cores, Intel(R) Xeon(R) Gold 5218 CPU @ 2.30GHz, 754 GB RAM
- GPU: NVIDIA(R) Tesla V100
- Python: 3.8.2
- PyPy: 7.3.9
- Clang: 13.0.1
- Codon: 0.15.0, commit 5480050b0ae3fb39b30e3a71df260ce5e91e5064
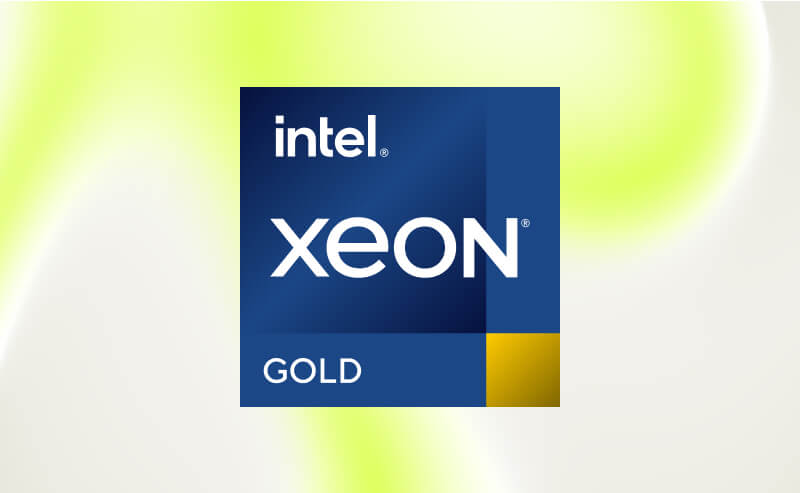
What our community is saying
On the CPU, the Python solution runs as fast as C++. The performance on the GPU is mind boggling. It does chunking, allowing one to specify a large N (e.g. 1 billion) and not worry about depleting GPU memory.
Your work is magnificent and inspirational. Codon is a glorious achievement and will influence the landscape of future human machine endeavors. Combining the high performance of native binaries and the intuitive natural cognitive ergonomics of Python is the best of both worlds. Big Ups to this team. Bravo.
This set of changes [to llama.py] adds ability to compile llama2.py with Codon. The result is a 74 X speedup!
For Enterprises
Support & Services
We offer enterprise licenses with support & services packages, custom solutions and more.
Exaloop Cloud
Run Codon in a fully managed and hosted environment. Scale on the cloud, no setup or dependency management needed.
Stay in the loop
Join our mailing list to stay updated on new features, product releases and announcements.